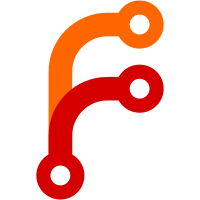
- Fix user serialization in TransactionPartnerUser - Remove null check for stickers in StickerSet - Fix nullable operator usage in ShippingQuery toArray method - Remove null-safe operator for traveler and watcher - Fix null-safe operator in PreCheckoutQuery.php - Fix null safe operator usage in PollAnswer.php - Refactor null checks in PassportData methods - Remove nullsafe operator from chat property - Refactor web_app handling in MenuButtonWebApp class - Fix conditional assignment in InputMediaVideo - Fix null coalescing and array mapping in InputMediaAudio - Fix redundant null coalescing in caption_entities mapping - Fix parsing of caption_entities field in InlineQueryResultPhoto - Fix potential unnecessary null coalescing in caption_entities - Fix redundant null coalescence check in reply_markup assignment - Fix redundant null coalescing in array_map calls - Refactor: remove unnecessary null coalescing operator - Fix conditional check for caption_entities assignment - Fix handling of input_message_content array conversion - Fix potential null pointer issue in InlineQuery class - Remove redundant null check in toArray method - Fix redundant null coalesce operator in photo mapping - Remove null safe operator for origin - Fix redundant null coalescing in array_map calls. - Fix optional chaining error in ChosenInlineResult.php - Remove null-safe operator in toArray() method - Fix null safe operator usage in ChatMemberRestricted - Fix null-safe operator usage in ChatMemberOwner.php - Fix null safe operator usage in ChatMemberMember.php - Fix nullable operator usage in ChatMemberLeft class - Fix null-safe operator in ChatMemberBanned serialization. - Fix user attribute null coalescing in ChatMemberAdministrator - Fix null-safe operator usage in ChatLocation conversion - Remove null safe operator in toArray() method - Fix nullable operator usage in ChatInviteLink serialization - Fix potential null pointer issue in ChatBoostSourcePremium. - Remove null safe operator in ChatBoostRemoved toArray method - Fix 'from' property serialization in CallbackQuery - Fix nullable operator usage on chat property - Remove redundant type check for associative array - Remove deprecated BotOld.php file
118 lines
No EOL
3.1 KiB
PHP
118 lines
No EOL
3.1 KiB
PHP
<?php
|
|
|
|
|
|
namespace TgBotLib\Objects\Inline;
|
|
|
|
use TgBotLib\Enums\Types\ChatType;
|
|
use TgBotLib\Interfaces\ObjectTypeInterface;
|
|
use TgBotLib\Objects\Location;
|
|
use TgBotLib\Objects\User;
|
|
|
|
class InlineQuery implements ObjectTypeInterface
|
|
{
|
|
private string $id;
|
|
private User $from;
|
|
private string $query;
|
|
private string $offset;
|
|
private ?ChatType $chat_type;
|
|
private ?Location $location;
|
|
|
|
/**
|
|
* Unique identifier for this query
|
|
*
|
|
* @return string
|
|
*/
|
|
public function getId(): string
|
|
{
|
|
return $this->id;
|
|
}
|
|
|
|
/**
|
|
* Sender
|
|
*
|
|
* @return User
|
|
*/
|
|
public function getFrom(): User
|
|
{
|
|
return $this->from;
|
|
}
|
|
|
|
/**
|
|
* Text of the query (up to 256 characters)
|
|
*
|
|
* @return string
|
|
*/
|
|
public function getQuery(): string
|
|
{
|
|
return $this->query;
|
|
}
|
|
|
|
/**
|
|
* Offset of the results to be returned, can be controlled by the bot
|
|
*
|
|
* @return string
|
|
*/
|
|
public function getOffset(): string
|
|
{
|
|
return $this->offset;
|
|
}
|
|
|
|
/**
|
|
* Optional. Type of the chat from which the inline query was sent. Can be either “sender” for a private chat
|
|
* with the inline query sender, “private”, “group”, “supergroup”, or “channel”. The chat type should be always
|
|
* known for requests sent from official clients and most third-party clients, unless the request was sent from
|
|
* a secret chat
|
|
*
|
|
* @return ChatType|null
|
|
*/
|
|
public function getChatType(): ?ChatType
|
|
{
|
|
return $this->chat_type;
|
|
}
|
|
|
|
/**
|
|
* Optional. Sender location, only for bots that request user location
|
|
*
|
|
* @return Location|null
|
|
*/
|
|
public function getLocation(): ?Location
|
|
{
|
|
return $this->location;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function toArray(): array
|
|
{
|
|
return [
|
|
'id' => $this->id,
|
|
'from' => $this->from->toArray(),
|
|
'query' => $this->query,
|
|
'offset' => $this->offset,
|
|
'chat_type' => $this->chat_type?->value,
|
|
'location' => $this->location?->toArray()
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public static function fromArray(?array $data): ?InlineQuery
|
|
{
|
|
if($data === null)
|
|
{
|
|
return null;
|
|
}
|
|
|
|
$object = new self();
|
|
$object->id = $data['id'];
|
|
$object->from = isset($data['from']) ? User::fromArray($data['from']) : null;
|
|
$object->query = $data['query'];
|
|
$object->offset = $data['offset'];
|
|
$object->chat_type = $data['chat_type'] ?? null;
|
|
$object->location = isset($data['location']) ? Location::fromArray($data['location']) : null;
|
|
|
|
return $object;
|
|
}
|
|
} |