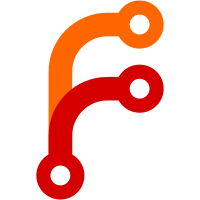
- Fix user serialization in TransactionPartnerUser - Remove null check for stickers in StickerSet - Fix nullable operator usage in ShippingQuery toArray method - Remove null-safe operator for traveler and watcher - Fix null-safe operator in PreCheckoutQuery.php - Fix null safe operator usage in PollAnswer.php - Refactor null checks in PassportData methods - Remove nullsafe operator from chat property - Refactor web_app handling in MenuButtonWebApp class - Fix conditional assignment in InputMediaVideo - Fix null coalescing and array mapping in InputMediaAudio - Fix redundant null coalescing in caption_entities mapping - Fix parsing of caption_entities field in InlineQueryResultPhoto - Fix potential unnecessary null coalescing in caption_entities - Fix redundant null coalescence check in reply_markup assignment - Fix redundant null coalescing in array_map calls - Refactor: remove unnecessary null coalescing operator - Fix conditional check for caption_entities assignment - Fix handling of input_message_content array conversion - Fix potential null pointer issue in InlineQuery class - Remove redundant null check in toArray method - Fix redundant null coalesce operator in photo mapping - Remove null safe operator for origin - Fix redundant null coalescing in array_map calls. - Fix optional chaining error in ChosenInlineResult.php - Remove null-safe operator in toArray() method - Fix null safe operator usage in ChatMemberRestricted - Fix null-safe operator usage in ChatMemberOwner.php - Fix null safe operator usage in ChatMemberMember.php - Fix nullable operator usage in ChatMemberLeft class - Fix null-safe operator in ChatMemberBanned serialization. - Fix user attribute null coalescing in ChatMemberAdministrator - Fix null-safe operator usage in ChatLocation conversion - Remove null safe operator in toArray() method - Fix nullable operator usage in ChatInviteLink serialization - Fix potential null pointer issue in ChatBoostSourcePremium. - Remove null safe operator in ChatBoostRemoved toArray method - Fix 'from' property serialization in CallbackQuery - Fix nullable operator usage on chat property - Remove redundant type check for associative array - Remove deprecated BotOld.php file
126 lines
No EOL
3.4 KiB
PHP
126 lines
No EOL
3.4 KiB
PHP
<?php
|
|
|
|
namespace TgBotLib\Objects;
|
|
|
|
use TgBotLib\Interfaces\ObjectTypeInterface;
|
|
|
|
class Venue implements ObjectTypeInterface
|
|
{
|
|
private Location $location;
|
|
private string $title;
|
|
private string $address;
|
|
private ?string $foursquare_id;
|
|
private ?string $foursquare_type;
|
|
private ?string $google_place_id;
|
|
private ?string $google_place_type;
|
|
|
|
/**
|
|
* Venue location. Can't be a live location
|
|
*
|
|
* @return Location
|
|
*/
|
|
public function getLocation(): Location
|
|
{
|
|
return $this->location;
|
|
}
|
|
|
|
/**
|
|
* Name of the venue
|
|
*
|
|
* @return string
|
|
*/
|
|
public function getTitle(): string
|
|
{
|
|
return $this->title;
|
|
}
|
|
|
|
/**
|
|
* Address of the venue
|
|
*
|
|
* @return string
|
|
*/
|
|
public function getAddress(): string
|
|
{
|
|
return $this->address;
|
|
}
|
|
|
|
/**
|
|
* Optional. Foursquare identifier of the venue
|
|
*
|
|
* @return string|null
|
|
*/
|
|
public function getFoursquareId(): ?string
|
|
{
|
|
return $this->foursquare_id;
|
|
}
|
|
|
|
/**
|
|
* Optional. Foursquare type of the venue.
|
|
* (For example, “arts_entertainment/default”, “arts_entertainment/aquarium” or “food/icecream”.)
|
|
*
|
|
* @return string|null
|
|
*/
|
|
public function getFoursquareType(): ?string
|
|
{
|
|
return $this->foursquare_type;
|
|
}
|
|
|
|
/**
|
|
* Optional. Google Places identifier of the venue
|
|
*
|
|
* @return string|null
|
|
*/
|
|
public function getGooglePlaceId(): ?string
|
|
{
|
|
return $this->google_place_id;
|
|
}
|
|
|
|
/**
|
|
* Optional. Google Places type of the venue. (See supported types.)
|
|
*
|
|
* @see https://developers.google.com/places/web-service/supported_types
|
|
* @return string|null
|
|
*/
|
|
public function getGooglePlaceType(): ?string
|
|
{
|
|
return $this->google_place_type;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function toArray(): array
|
|
{
|
|
return [
|
|
'location' => $this->location->toArray(),
|
|
'title' => $this->title,
|
|
'address' => $this->address,
|
|
'foursquare_id' => $this->foursquare_id,
|
|
'foursquare_type' => $this->foursquare_type,
|
|
'google_place_id' => $this->google_place_id,
|
|
'google_place_type' => $this->google_place_type,
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public static function fromArray(?array $data): ?Venue
|
|
{
|
|
if($data === null)
|
|
{
|
|
return null;
|
|
}
|
|
|
|
$object = new self();
|
|
$object->location = isset($data['location']) ? Location::fromArray($data['location']) : null;
|
|
$object->title = $data['title'];
|
|
$object->address = $data['address'];
|
|
$object->foursquare_id = $data['foursquare_id'] ?? null;
|
|
$object->foursquare_type = $data['foursquare_type'] ?? null;
|
|
$object->google_place_id = $data['google_place_id'] ?? null;
|
|
$object->google_place_type = $data['google_place_type'] ?? null;
|
|
|
|
return $object;
|
|
}
|
|
} |